Introduction.
Web development continues to evolve to achieve a more interactive and responsive UX. The evolutionary process is described, starting with server-side rendering (SSR), followed by Ajax, single page applications (SPA), and virtual DOM.
Server-side rendering (SSR): ~2000
In early web development, all content was generated on the server side and sent to the client (browser) (=SSR).
When the user clicks on the link, a request for a new page is sent to the server, which generates a new HTML page and sends it back to the client.
Since a static HTML file is displayed, any changes to the data will not be reflected unless the file is reloaded.
Advantages: Faster initial display speeds, as fully rendered pages are served promptly to the user.
Cons: Page transitions can be slow because all HTML must be reloaded from the server each time the user moves to a different page. The entire screen goes blank for a moment, during which time the user cannot operate.
Emergence of Ajax → SPA spread: 2000~2010
Asynchronous JavaScript and XML ( Ajax ) was introduced in the early 2000s. Ajax is a technology that uses JavaScript to communicate asynchronously with a server to dynamically update portions of a web page. This allowed users to have a smoother, more interactive web experience.
Google Maps is one example of this technology that has become widely known. The entire screen does not go blank (SSR) every time you move the display portion of the map.
Advantages: only part of the page can be updated (no page reloading); you do not have to wait until one process is finished to do another.
Cons: Complex Ajax applications can be difficult to manage.
How Ajax works: XMLHttpRequest / JavaScript / DOM
Ajax combines technologies and concepts such as XMLHttpRequest, JavaScript, XML, JSON, and DOM.
However, as the name suggests, the XMLHttpRequest
object was initially intended primarily to retrieve XML data, but it can handle any type of data and does not necessarily need to be in XML format. It is also possible to pass non-XML data (e.g., JSON) in the form of a response from the server, so it does not need to be in XML format as well.
Initially, Ajax was primarily used to receive and send data in XML format, but JSON is now more widely adopted; XML is still available as an option but is not required for Ajax communication. In other words, despite its name, Ajax (Asynchronous JavaScript and XML) is not limited to XML, so the term XML may be a mismatch at this time.
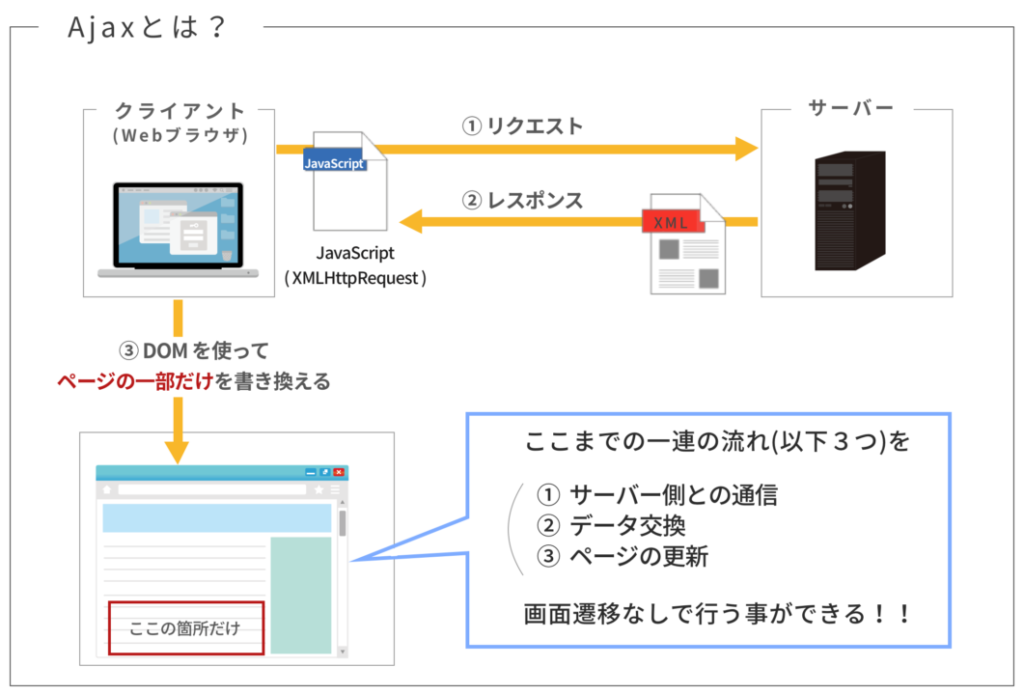
1. triggering of events
When a user performs an action (e.g., button click) on a web page, the event is triggered.
Asynchronous requests to the server
After the event occurs, use JavaScript to create an XMLHttpRequest
object. This object is used to send a request asynchronously to the server. The request specifies the information required by the user and the expected response format (JSON, XML, etc.).
3. request processing on the server
The server receives the request and performs the necessary processing. During this time, other operations can be performed on the client side, and the user can continue to interact with the page without waiting.
4. sending a response
When processing is completed on the server, the results are sent to the client in JSON, XML, or other formats (response).
Since communication to the server uses the XMLHttpRequest
object, it seems that only XML data can be received, but it is possible to receive data other than XML (e.g., JSON).
XML stands for Extensible Markup Language, a markup language similar to HTML, but unlike HTML, it allows tag names to be freely configured, making it ideal for managing the exchange of multiple processes.
5. update DOM
Client-side JavaScript updates the DOM (Document Object Model) with the received response. In this step, only a portion of the page is rewritten with the new data. This eliminates the need to reload the entire page, providing a smooth and seamless experience for the user.
The DOM is a mechanism that allows documents to be manipulated and used from programs (such as JavaScript) by representing HTML and XML in a tree structure model of objects.
As for the DOM, this explanation is easy to understand.
https://note.och.co.jp/n/nc24a672494c3
In the code below, when the user clicks on the “Load Data” button, script.js
loads data from data.txt
asynchronously and inserts that data in a div
tag with the ID dataContainer
.
This process is performed without reloading the entire page, so the user can continue to use other parts of the page. Also, only the content in the dataContainer
needs to be updated, not the entire page.
<title>Ajax Example</title>
<button id="loadButton">Load data</button>
<div id="dataContainer">Data will be displayed here</div>
// script.js
document.getElementById('loadButton').addEventListener('click', function() {
var xhr = new XMLHttpRequest(); // Create XMLHttpRequest object.
xhr.open('GET', 'data.txt'); // Set up a GET request to retrieve data from data.txt
xhr.onreadystatechange = function() {
if (xhr.readyState == 4 && xhr.status == 200) {
document.getElementById('dataContainer').innerHTML = xhr.responseText; // Insert response into dataContainer; DOM is used.
}
};
xhr.send(); // Send request
});
For more information on how Ajax works, click here.
https://pikawaka.com/word/ajax
Single Page Application (SPA)
With the development of Ajax, web applications have further evolved and the concept of Single Page Applications (SPA) has emerged.
As the name suggests, SPA refers to a web application that switches content on a single page.
After first obtaining the screen (HTML), the screen can be rewritten by JavaScript to build an application, albeit a single page.
First-page load
- In SPA, all core assets of the application (HTML, JavaScript, and CSS) are loaded onto the client at once during the first access. Specifically, the JavaScript and CSS bundler combines multiple JavaScript, and CSS files into one file at build time.
- Unlike the traditional multi-page application (MPA) approach, it eliminates the need to load the necessary resources from the server each time there is a transition between pages.
Transition between pages
- Fetch only necessary data using JavaScript (asynchronous communication including Ajax) and dynamically update the view on the client side.
- It does not mean that a single page = a single URL. URLs can be manipulated with JavaScript (using React Router, Vue Router, etc. for front-end frameworks such as React and Vue.js), so even if the URL is changed, page reloading does not occur and a single The page can remain a single page even if the URL is changed. From the user’s perspective, it appears as if the page is transitioning in the same way as MPA, but it is actually a single page.
Advantage: Smooth page transitions
Cons: May take a long time to load, which can affect SEO initially
Introduction of Virtual DOM: 2013
Ajax enabled asynchronous communication, allowing data to be retrieved from the server and specific DOM elements to be updated without reloading the entire page.
However, this process often increased the complexity of the JavaScript code and made it difficult to keep the DOM and data consistent, especially in large applications.
To solve the issue of code complexity, Facebook released a library called React in 2013, which took the approach of a virtual DOM as a means of synchronizing the DOM and retrieved data.
When the state of a component changes, React rebuilds the virtual DOM and calculates the difference from the previous virtual DOM (called Reconciliation). Only the necessary parts are updated by applying the difference to the actual DOM.
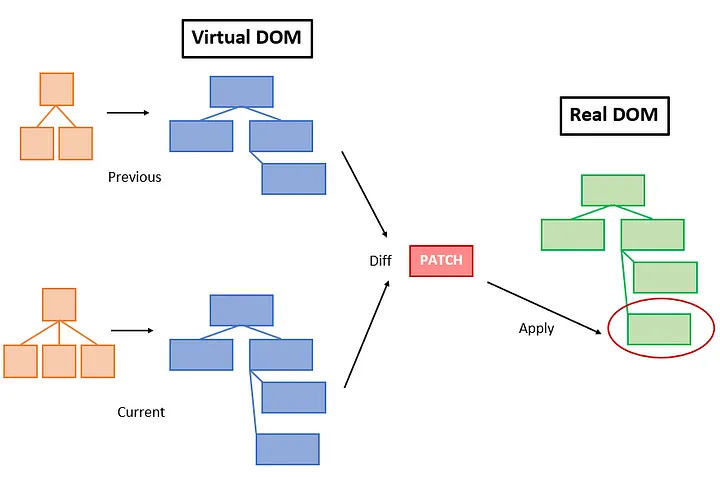
virtual DOM
- A structure that simulates the DOM in memory space, etc. When modified, data in the memory space is rewritten.
- Although it has DOM in its name, it is not an object that is directly displayed in the browser. It is an ordinary JavaScript object.
- By detecting updates to portions of the virtual DOM and synchronizing them with the DOM, only the differences can be detected. This process is automatic, and the developer does not need to individually specify which DOM elements should be updated.
https://react.dev/reference/react-dom/components
Difference between real DOM and virtual DOM
Example: Counter
Real DOM
<title>Counter Example</title>
<div id="app">
<p id="counter">0</p>
<button type="button" id="increment">+1</button>
</div>
let count = 0;
const btn = document.getElementById('increment');
const counterElement = document.getElementById('counter');
btn.addEventListener('click', () => {
count++;
counterElement.innerText = count;
});
The following disadvantages
- A mixture of UI and logic: UI (HTML) and logic (JavaScript) are mixed because DOM elements are directly referenced and manipulated in JavaScript.
- Dual management of state: The state of the counter is managed dually in a JavaScript variable (count) and in the DOM (text
in <p id="counter">0</p>
). Each time the count increases, the two states must be manually synchronized. - Event Listener Settings: Event listeners for buttons must be set manually.
- Cost of DOM manipulation: Each time a counter is updated, the DOM must be manipulated directly. This can impact performance, especially in large applications with many DOM elements.
If the above is written in REACT
import React, { useState } from 'react';
export default function Counter() {
const [count, setCount] = useState(0); // State management with React.
const increment = () => {
setCount(prevCount => prevCount + 1);
};
// UI is automatically updated based on status
return (
<div>
<p>{count}</p>
<button type="button">+1</button>
</div>
);
}
- Simplified state management: counter state is managed using the
useState
hook, clearly separating UI and logic.count is
managed as a React state, and the UI (<p>{count}</p>)
always reflects this state. - Declarative update of UI: When the state of the message changes, the virtual DOM detects this change and only the necessary UI parts are updated efficiently.
- Simplified event handler: The event handler is specified directly through the button’s
onClick
property. This eliminates the need to reference DOM elements directly and simplifies the code.
In React, the developer does not directly specify which DOM elements to update but rather defines “rules” such as “this is how the UI should look when the state changes, ” and React’s internal mechanism is in charge of specific DOM operations.
<p>{count}</p>
In conventional DOM manipulation using JavaScript, the developer directly specifies specific commands such as “When this button is clicked, update the innerText of this
document.getElementById('counter').innerText = count;
The virtual DOM tree corresponding to this component can be thought of as having the following structure
div
├── p
│ └── "Current value of count"
└── button
└── "+1"
Here, "current value of count"
is a text node that depends on the count
state. When the user clicks the button, the setCount
function is called and the count
state is updated.
React detects this change and generates a new virtual DOM tree with the new count
value. This internal virtual DOM tree cannot be touched directly and is managed by React abstraction. The old and new virtual DOM trees are then compared, and only the parts that have changed (in this case, the text in the
Challenges and Developments in Virtual DOM: Incremental, Fiber, and Svelte
The virtual DOM is not perfect and has the following issues.
Additional memory usage
- The virtual DOM is an in-memory representation of the actual DOM and may use more memory because it holds virtual nodes for all parts of the application’s UI structure.
Delay in the initial rendering
- Because the virtual DOM must be compared to the actual DOM, the first rendering may take some time to synchronize with the actual DOM.
Performance overhead
- The virtual DOM requires additional processing, such as comparison with the actual DOM and difference calculations. This may result in performance overhead in some cases.
Several methods have been mentioned as developments of the virtual DOM that solve the above issues.
Incremental DOM (2016?)
- Incremental DOM focuses on updating only those areas that need to be changed.
- Developed by Google and adopted by Angular.
- Advantages: reduced memory usage, since changes are applied on the fly as they are made to the DOM, so there is no need to keep a copy of the entire DOM tree.
- Disadvantage: Because changes are applied directly to the actual DOM, processing time may be required for applications with many changes or large applications, as the DOM must be updated each time.
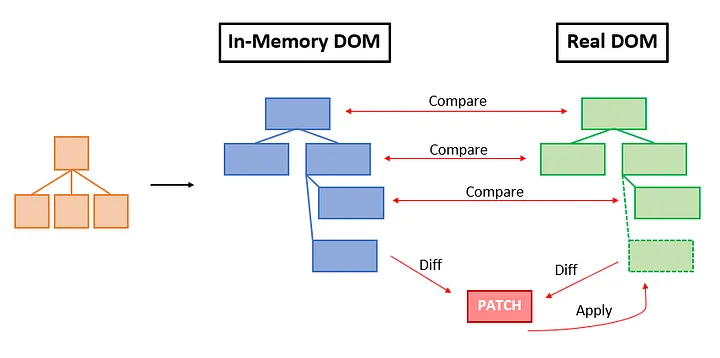
Explanation of the difference between Virtual DOM and Incremental DOM here
https://blog.bitsrc.io/incremental-vs-virtual-dom-eb7157e43dca
Fiber (2017)
- Introduced in React 16.
- Divide components into new work units called “fibers”.
- Fiber improves application responsiveness by breaking the update work into smaller pieces for each component and allowing React to suspend and resume these tasks as needed.
For more information
https://zenn.dev/arranzt/articles/01807d1b3d2fc1
Svelte (2016).
- Tracks change at compile time and directly generates optimal DOM operations. Because changes are reflected without the virtual DOM, runtime overhead is reduced and faster applications can be realized.
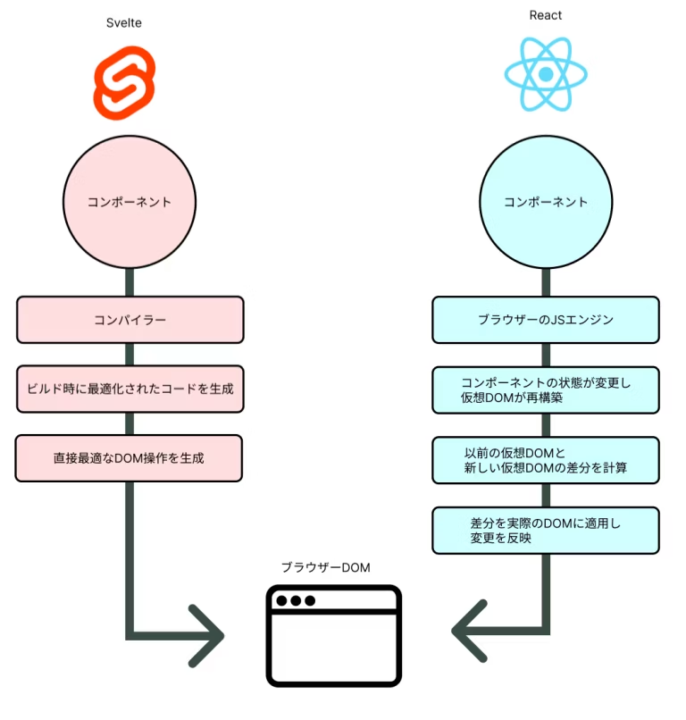
PWA (Progressive Web Apps): 2015
With the proliferation of mobile and the growing need for offline use by users (e.g., on the subway) and a fast app experience, Google began advocating a system called PWA in about 2015.
A PWA is a mechanism that allows a website to be used like a native application.
- Websites that implement PWA can use the same functions as native apps, such as working offline and sending push notifications. Unlike native apps, there is no need to go through an app store and no installation is required.
- Examples: Twitter, YouTube, Spotify, Pinterest, Uber, Suumo, Nikkei, etc.
- Offline functionality: Accessible even when offline using a technology called Service Worker, which acts as a proxy between the browser and the network and can cache data. Taking browser caching a step further, cache management via Service Worker allows developers to specify in detail which resources to cache and when and how to update them. So apps can function even when offline and provide a more controlled experience for users.
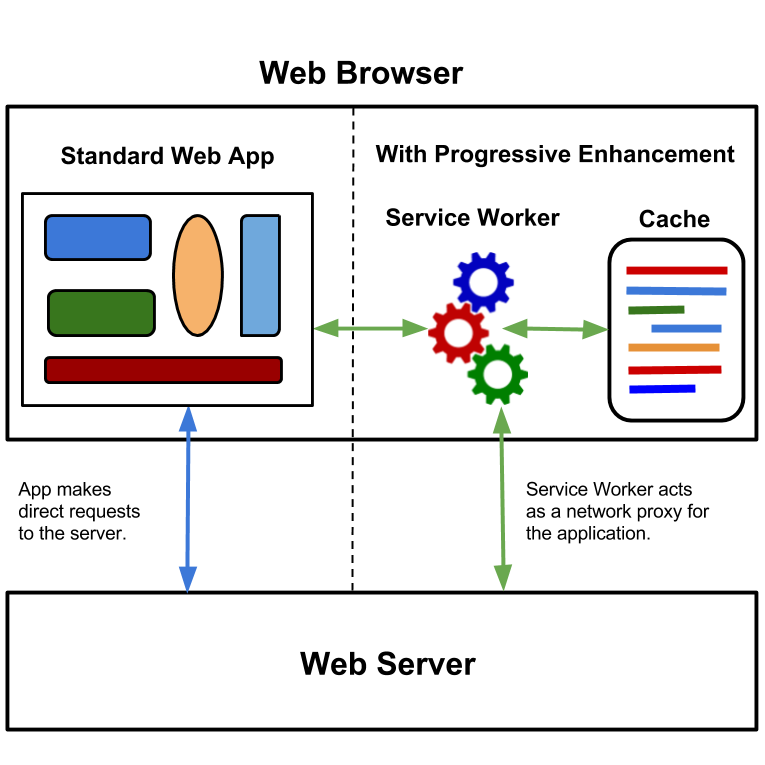
summary
- Server-side rendering (SSR): Before 2000, all content was generated on the server and sent to the client.
- The emergence of Ajax and SPA: From the early 2000s to around 2010, single-page applications (SPAs) emerged as the technology to update only a portion of a page through asynchronous communication using Ajax became popular.
- Introduction of Virtual DOM: In 2013, Facebook released React, which introduced an efficient method of updating UI using the virtual DOM.
- PWA: In 2015, Google promoted PWAs, providing a mechanism to allow websites to be used like native apps.
コメント